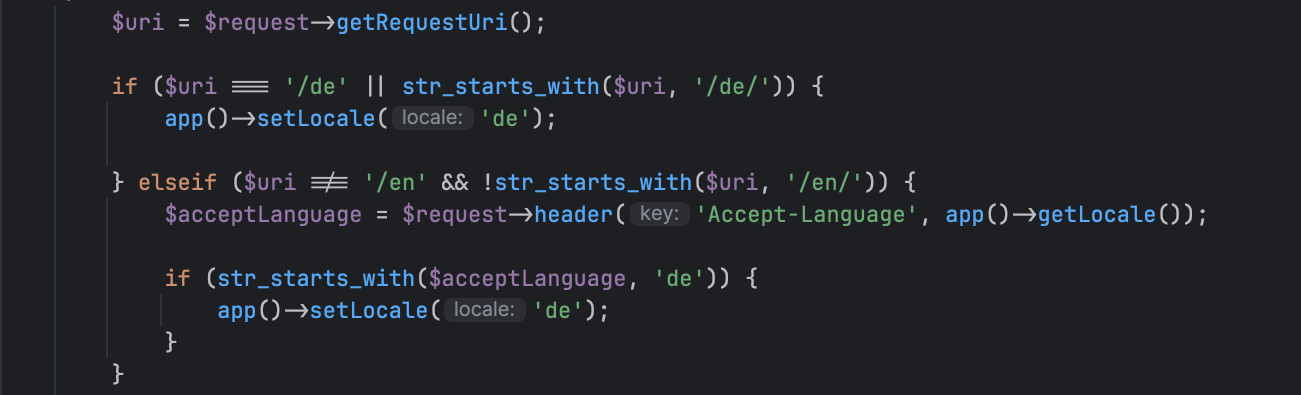
Multiple languages in Laravel
It is not unusual for customers to require multilingual applications or sites. Some CMS' offer support for multiple languages out-of-the-box. However, if a special solution consisting of application and website is required, it often makes sense to develop from scratch because of complexity. Laravel, as one of the most widely used and most popular PHP frameworks, offers the most important building blocks for applications and websites without the need of additional packages or plugins. The feature of multiple languages can be easily implemented using Laravel's middleware.
In the following example, we only want a German and an English version of the website. As mentioned before, we use middleware to determine the language of the current request.
The middleware is simply generated by using Laravel's artisan command...
$ php artisan make:middleware SetLocaleMiddlewareINFO Middleware [app/Http/Middleware/SetLocaleMiddleware.php] created successfully.
...and registered in bootstrap/app.php:
->withMiddleware(function (Middleware $middleware) { // ... $middleware->append(App\Http\Middleware\SetLocaleMiddleware::class); // ...})
Now we feed the middleware with instructions so that the desired language can be determined. To do this, the following code is inserted into our new SetLocaleMiddleware.php. It is important to note that English is used as the default language in the Laravel configuration.
public function handle(Request $request, Closure $next): Response{ $uri = $request->getRequestUri(); if ($uri === '/de' || str_starts_with($uri, '/de/')) { app()->setLocale('de'); } elseif ($uri !== '/en' && !str_starts_with($uri, '/en/')) { $acceptLanguage = $request->header('Accept-Language', app()->getLocale()); if (str_starts_with($acceptLanguage, 'de')) { app()->setLocale('de'); } } return $next($request);}
In order to be forwarded directly to the appropriate language when visiting the start page of the site, we change our routes/web.php as follows:
Route::get('/', function () { return response()->redirectTo(app()->getLocale());});
When visiting the base URL, the locale determined and stored in our middleware is retrieved and visitors are forwarded directly to the appropriate language as specified by their browser's request header Accept-Language. If the language was not found in the middleware, visitors are automatically redirected to the default language. In this case, English.
With this setup, translations can now also be easily output via __(). Whenever access to the current language is required, it can be retrieved with app()->getLocale().
Have fun translating!